Conquering Cocoa Errors: A Developer’s Guide
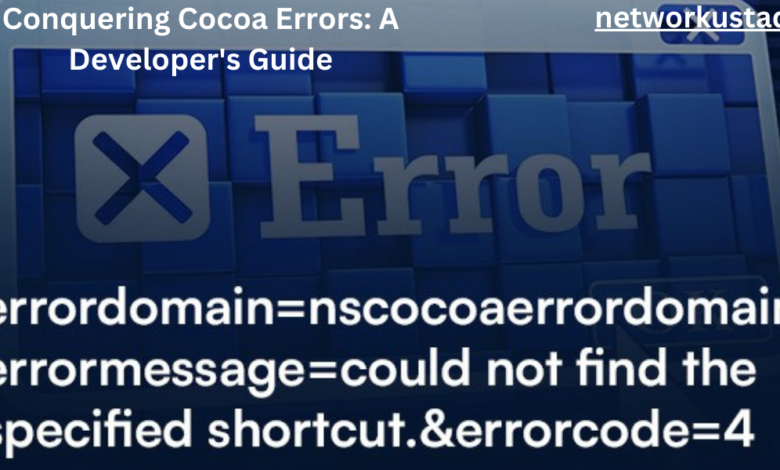
Navigating the complexities of error handling in Cocoa can be daunting for developers. This guide aims to demystify the process. It gives tips for handling errors with the NSError class in Cocoa and Objective-C. It covers common fixes for NSCocoaErrorDomain errors.
Understanding NSError
What is NSError?
- NSError is a key class in the Foundation framework.
It is designed to hold error information in a structured way.
Rewritten
in active voice: The system is designed to store error information in a structured manner. - An NSError object has three critical pieces of information. It has a status code, an error domain, and a user info dictionary. The dictionary gives more context.
- Error Domains: Each NSError object has a status code. It is in a specific error domain to avoid overlap and confusion.
Key Features of NSError
- User Info Dictionary: This dictionary can hold key-value pairs. They offer extra context about the error. It can also include another NSError object representing an underlying error.
- Objects pass NSError objects between contexts without obstruction. This helps with error handling across an app.
- Error Codes and Descriptions: The table is extensive. It has error codes and descriptions for categories like FTP, HTTP, SSL, and more.
Best Practices for Managing NSError
Defining Error Domains and Codes
- Best practices involve managing error domains. They handle error codes and user info dictionaries with unified control. You should do this across the whole application.
- In header files, define constants in a header file. Include the header file wherever needed.
Customizing NSError
- You can subclass NSError. Do this to change the localized description or other properties.
Error Handling in Cocoa
- Apple’s Error Handling Programming Guide for Cocoa has detailed articles. They cover error objects, domains, and codes. It also covers using and creating error objects.
- The guide also covers error responders and recovery. These are available only in the Application Kit (OS X).
Common Cocoa Errors and Remedies
Table of Common Error Domains and Codes
Error Domain | Description |
NSMachErrorDomain | Errors related to Mach system calls |
NSPOSIXErrorDomain | Errors related to POSIX system calls |
NSOSStatusErrorDomain | Errors related to OSStatus codes |
NSCocoaErrorDomain | General Cocoa framework errors |
By using these domains and their error codes. Developers can better find and fix issues in their applications.
NSCocoaErrorDomain Errors
- File write errors: Errors like “NSFileWriteUnknownError” occur when trying to save a document. Error details reside in the NSUnderlyingErrorKey property for reference.
- Delete Action Errors: NSCocoaErrorDomain Code=4 occurs during recursive directory deletion attempts. Locked files or files with unusual characters may cause this error.
Troubleshooting Steps
- Identify Source of Error: Investigate the user info dictionary for underlying error information.
- Handle Locked Files. Make sure to handle locked files before performing actions like deletion.
Error Handling in Swift
Integrating with Objective-C
- You need to call Objective-C methods with NSError ** parameters. Swift can cut the visible error handling code.
- Swift is supposed to make error handling simpler. But, it can also make basic code more tedious without big benefits. Yet, Swift has its own powerful error handling mechanisms. They use try, catch, throw, and Result. These options offer more flexibility and control.
Frequently Asked Questions (FAQ)
Q1: How do I access the underlying error information within an NSError?
A: The userInfo dictionary of an NSError object can contain an entry with the key NSUnderlyingErrorKey. The value associated with this key is often another NSError object that provides more specific details about the root cause.
Q2: What is the purpose of defining custom error domains and codes?
You have the power to define your own custom error domains and codes within the applications you build.
. This enhances code clarity and organization. This practice makes it easier to find the source of errors. It lets you apply specific handling logic.
Q3: Can I use Swift’s error handling features when working with Objective-C code?
A: Yes, Swift seamlessly integrates with Objective-C’s error handling patterns. When calling Objective-C methods, they return errors via NSError. Swift adapts them to its own error handling.
Conclusion
Managing errors in Cocoa apps requires a deep understanding of the ‘NSError’ class. By using best practices and Apple’s tools, developers can make robust apps. The apps can handle errors gracefully and give users a better experience.